Adding An ArcGIS Item Dataset¶
Problem¶
You want to use Boson to connect to a data source which is available as a Feature, Image, or Map Service with an Item ID in ArcGIS Online or ArcGIS Enterprise.
Solution¶
In this example, we will be using a fire perimeter layer from the HIFLD Open Data Catalogue. This service has the item id: c7d3a41994a14277836d3c1cd514a2e3. This layer is an ArcGIS feature service with polygons showing the perimeters of fires in the US from 2000 to 2013. The layer is available here. Because this is a public layer, we can access it without any credentials.
Note
You will need to install the ArcGIS Python API to run this example. You can do this by running pip install arcgis
.
Setup¶
First to do some initial setup. We start by importing geodesic:
>>> import geodesic
If you haven’t yet, you will need to authenticate geodesic using the following command:
>> geodesic.authenticate()
This process is covered in more detail in the Quickstart Guide.
We need to set the active project to ensure that our dataset is saved to the correct project. We do this using the uid of our desired project. If you do not know the uid, you can fetch a dictionary of existing project that you can access by running geodesic.get_projects()
. Once you have the uid, you can set your active project like so:
>>> geodesic.set_active_project('cookbook-examples')
Creating The Provider¶
The geodesic python API provides a method, geodesic.Dataset.from_arcgis_layer()
which makes adding an ArcGIS Layer dataset extremely straightforward. To add our fire perimeter dataset, we simply run:
>>> ds = geodesic.Dataset.from_arcgis_item(
name='fire-perimeters',
arcgis_instance='https://www.arcgis.com',
item_id='c7d3a41994a14277836d3c1cd514a2e3'
)
>>> ds.save()
This will create a new dataset in our active project called ‘fire-perimeters’ and save it to the project.
Note
In this case we are using a public source on www.arcgis.com. This is the default argument
so if using this instance you dont need to specify it, but is done here as an example. If you are using an ArcGIS Enterprise instance,
you will need to provide the url of your instance as the arcgis_instance
argument.
Testing The Provider¶
Now to run a quick test to ensure that the provider is working. Let’s search run a simple search to check that features are returned
>>> ds.search()
This should return the first ten features from the ArcGIS Layer. If you are running in a jupyter notebook, this should appear in a widget like so:
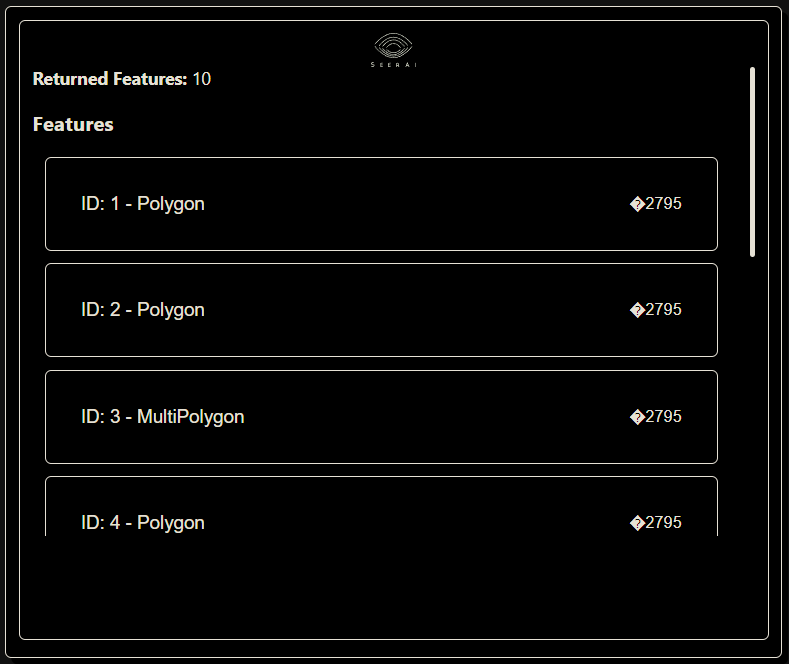
You can expand the individual features on the list to check that the correct data fields are included:
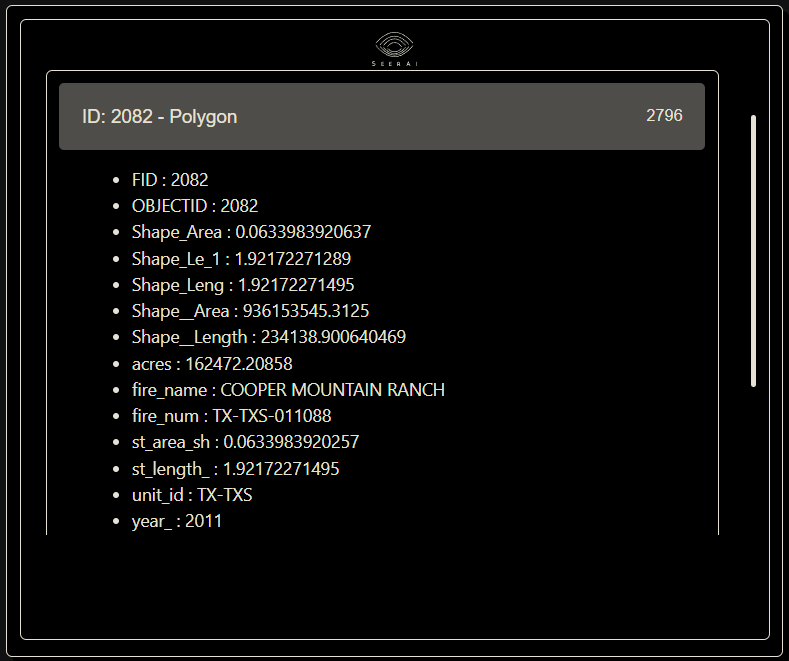
If you are missing the dependencies required to generate the jupyter widgets, or are not running in a notebook at all, the ds.search() method will return a dict containing the same information.
As a slightly less trivial example, let’s search for fires from the year 2011, that took place in Texas, and were larger than 150k acres:
>>> from geodesic import cql
>>> feats = ds.search(
limit=10,
filter=cql.CQLFilter.logical_and(
cql.CQLFilter.eq('year_', '2011'),
cql.CQLFilter.gte('acres', 150000),
cql.CQLFilter.eq('unit_id', 'TX-TXS')
)
)
>>> feats
This should return three features:
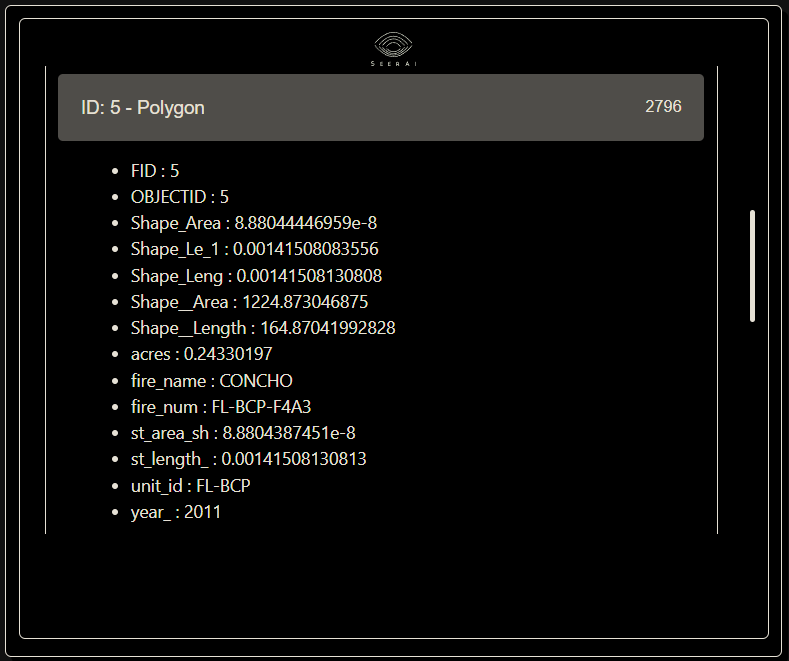
Finally, if you have installed the relevant dependencies, you can use the geodesic mapping utilities to visualize these features on a map using the following lines:
>>> from geodesic import mapping
>>> m = mapping.Map()
>>> m.add_feature_collection('fires', feats)
>>> m
If you use the map to navigate to the area of Texas, you should see the three features from our search:
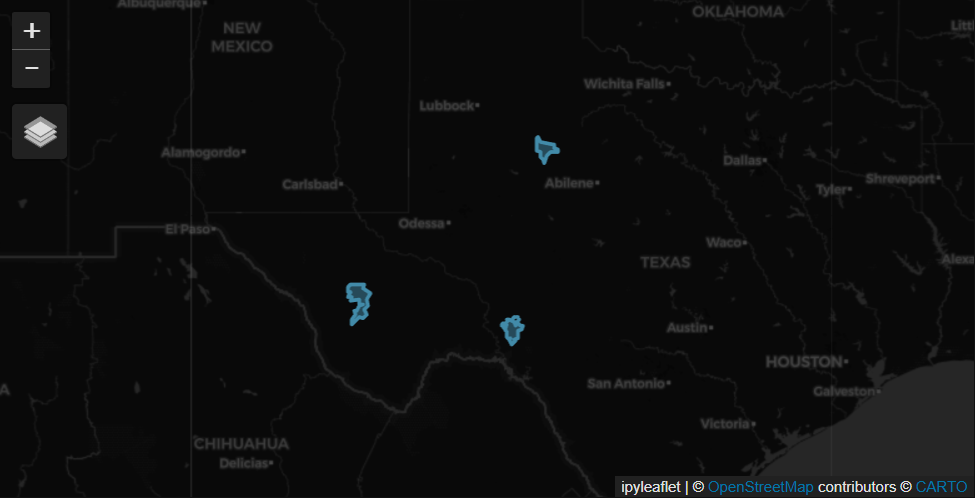