Account Management#
To use the Geodesic API you must first authenticate your account with our the platform. This can be done either from Python or from the command line.
CLI Authentication#
$ geodesic authenticate
geodesic authenticate
To authorize access needed by Geodesic, open the following URL in a web browser and follow the instructions.
If the web browser does not start automatically, please manually browse the URL below.
A browser window should open and you will see the following screen.
{
"api_key": "XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX"
}
Note
Please check if your browser displays the above JSON as raw or parsed text. If it’s parsed, you’ll need to copy the value("XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX"
) from the key. If it’s raw, you can copy everything between the {}
.
Python Authentication
Python authentication can be done either in the python interpreter or a Jupyter environment.
import geodesic
geodesic.authenticate()
Depending on your IDE, you may be prompted to enter the API key in various locations in the program.
Visual Studio Code
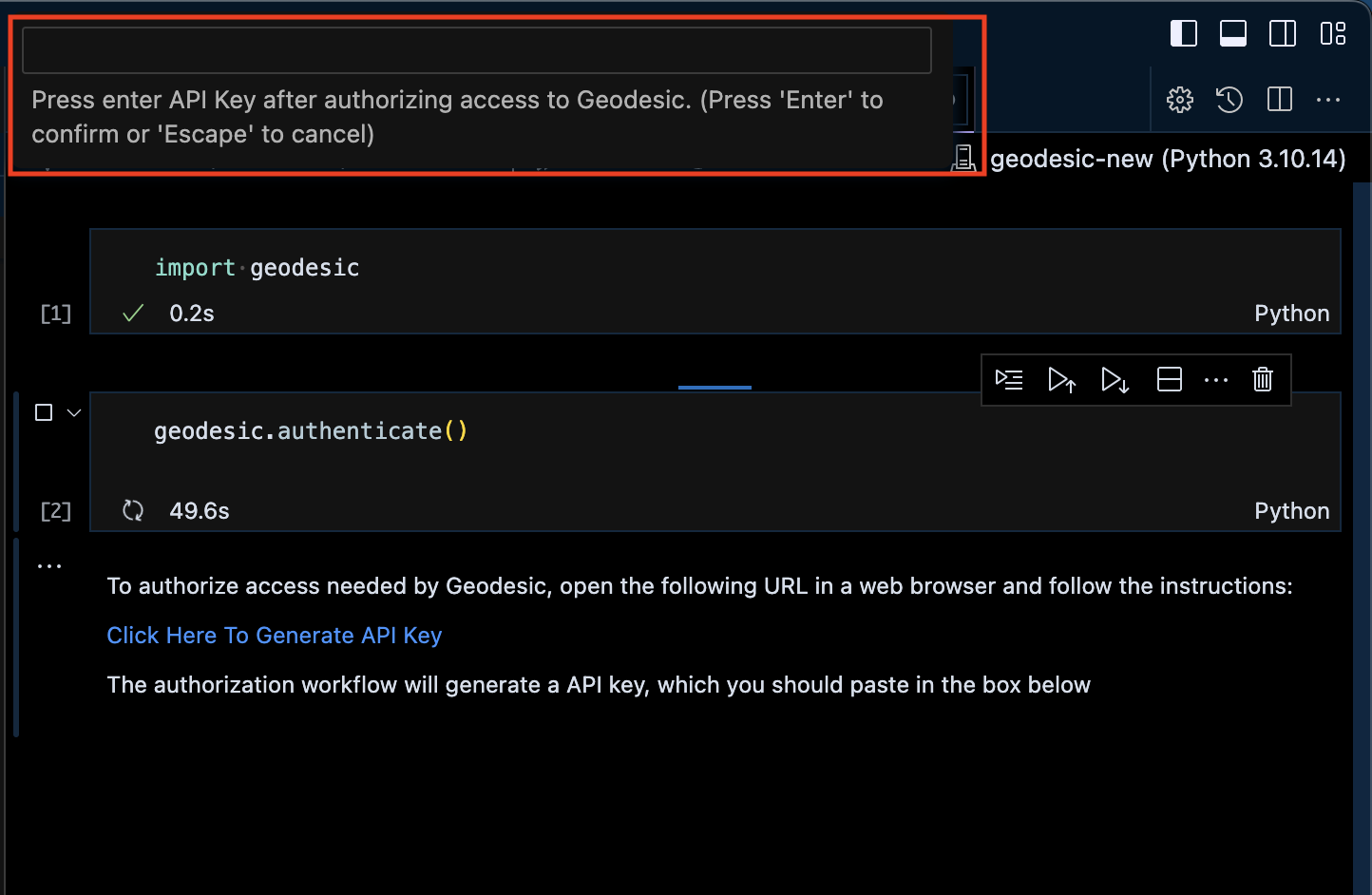
Jupyter Lab
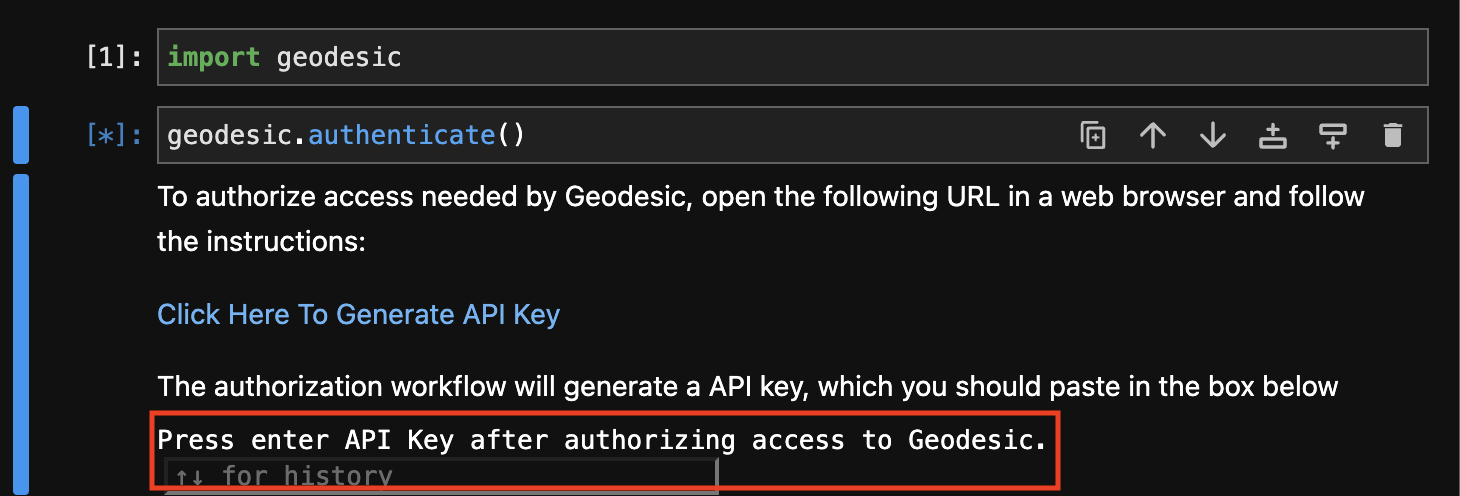
Note
You will not need to log in each time you use the API. The credentials stored are long lived. If you are worried that you may have copied the key incorrectly you can check the stored credentials in ~/.config/geodesic/config.json
file.
Your Account information#
When you access Geodesic, the API reaches out to an external identity provider to get a token to interact with the backend. That token carries some information about you and your current permissions level, but also we have a backend service that adds to and customizes that information. You can get your user profile using the built-in geodesic.account.user.myself()
function. You are uniquely identified in the system by the subject
field.
u = geodesic.myself()
dict(u)
{'subject': 'auth0|611c3d206a08f0006c8a9290',
'first_name': '',
'last_name': '',
'middle_name': '',
'alias': 'gcp-headless@seerai.space',
'email': 'gcp-headless@seerai.space',
'avatar': 'https://s.gravatar.com/avatar/ff1b38649bdb3d54918f07a81ba0b036?s=480&r=pg&d=https%3A%2F%2Fcdn.auth0.com%2Favatars%2Fgc.png',
'pronouns': '',
'bio': ''}
You can edit all of this information directly.
u.first_name = "Geodesic"
u.middle_name = "API"
u.last_name = "User"
u.alias = "Headless User"
u.pronouns = "it/its"
u.bio = "I'm a robot that manages some internal stuff for SeerAI. Will you be my friend?"
u.save()
dict(u)
{'subject': 'auth0|611c3d206a08f0006c8a9290',
'first_name': 'Geodesic',
'last_name': 'User',
'middle_name': 'API',
'alias': 'Headless User',
'email': 'gcp-headless@seerai.space',
'avatar': 'https://s.gravatar.com/avatar/ff1b38649bdb3d54918f07a81ba0b036?s=480&r=pg&d=https%3A%2F%2Fcdn.auth0.com%2Favatars%2Fgc.png',
'pronouns': 'it/its',
'bio': "I'm a robot that manages some internal stuff for SeerAI. Will you be my friend?"}
You can check permissions and roles.
print(f'permissions = {u.get_permissions}, roles = {u.get_roles}')
permissions = ['spacetime:read', 'spacetime:write', 'tesseract:read', 'tesseract:write', 'entanglement:read', 'entanglement:write'], roles = ['user']