Adding An ArcGIS Service Dataset#
Problem#
You want to use Boson to connect to a data source which is available as a Feature, Image, or Map Service in ArcGIS Online or ArcGIS Enterprise.
Solution#
In this example, we will be using a Coastal Barrier Resources System (CBRS) Boundaries layer from the HIFLD Open Data Catalogue. This layer is an ArcGIS Map service featuring polygons that represent the Coastal Barrier Resources System, a network of protected coastal areas. These coastal barriers play a vital role as buffers, shielding inland regions and properties from severe flood damage caused by coastal storms. The layer is available here. Because this is a public layer, we can access it without any credentials.
Setup#
To begin we start by importing geodesic:
import geodesic
If you haven’t yet, you will need to authenticate geodesic using the following command:
geodesic.authenticate()
This process is covered in more detail in the Getting Started Guide.
We need to set the active project to ensure that our dataset is saved to the correct project. We do this using the uid of our desired project. If you do not know the uid, you can fetch a dictionary of existing project that you can access by running geodesic.account.projects.get_projects()
. Once you have the uid, you can set your active project like so:
geodesic.set_active_project('cookbook-examples')
If you need to create a new project the process is detailed in Geodesic Projects page.
Creating The Provider#
The geodesic python API provides a method, geodesic.boson.dataset.Dataset.from_arcgis_service()
which makes adding an ArcGIS Service dataset extremely straightforward.
This method supports adding Feature, Map and Image services.
ds = geodesic.Dataset.from_arcgis_service(
name='cbrs-boundaries',
url='https://cbrsgis.wim.usgs.gov/arcgis/rest/services/FEMA/CBRS_Prohibitions/MapServer',
layer_id=0
)
ds.save()
This will create a new dataset in our active project called ‘cbrs-boundaries’ and save it to the project.
Testing The Provider#
Now to run a quick test to ensure that the provider is working. Let’s search run a simple search to check that features are returned
ds.search()
This should return the first ten features from the ArcGIS Layer.
Additionally, we can pass a bounding box to the search method to filter the results to only those features that intersect with the bounding box. For example, to search for features that intersect with a bounding box around north shore of Massachusetts, we can use the following code:
bbox_ma = [-70.989645,42.548461,-70.558432,42.881432]
feats = ds.search(
limit=None,
bbox=bbox_ma
)
feats
This should return thirteen features:
Finally, if you have installed the relevant dependencies, you can use the geodesic mapping utilities to visualize these features on a map using the following lines:
from geodesic import mapping
m = mapping.Map(center = [42.725610,-70.774162], zoom = 10)
m.add_feature_collection('coastal barriers', feats)
m
If you use the map to navigate the north shore of Massachusetts, you should see the thirteen features from our search:
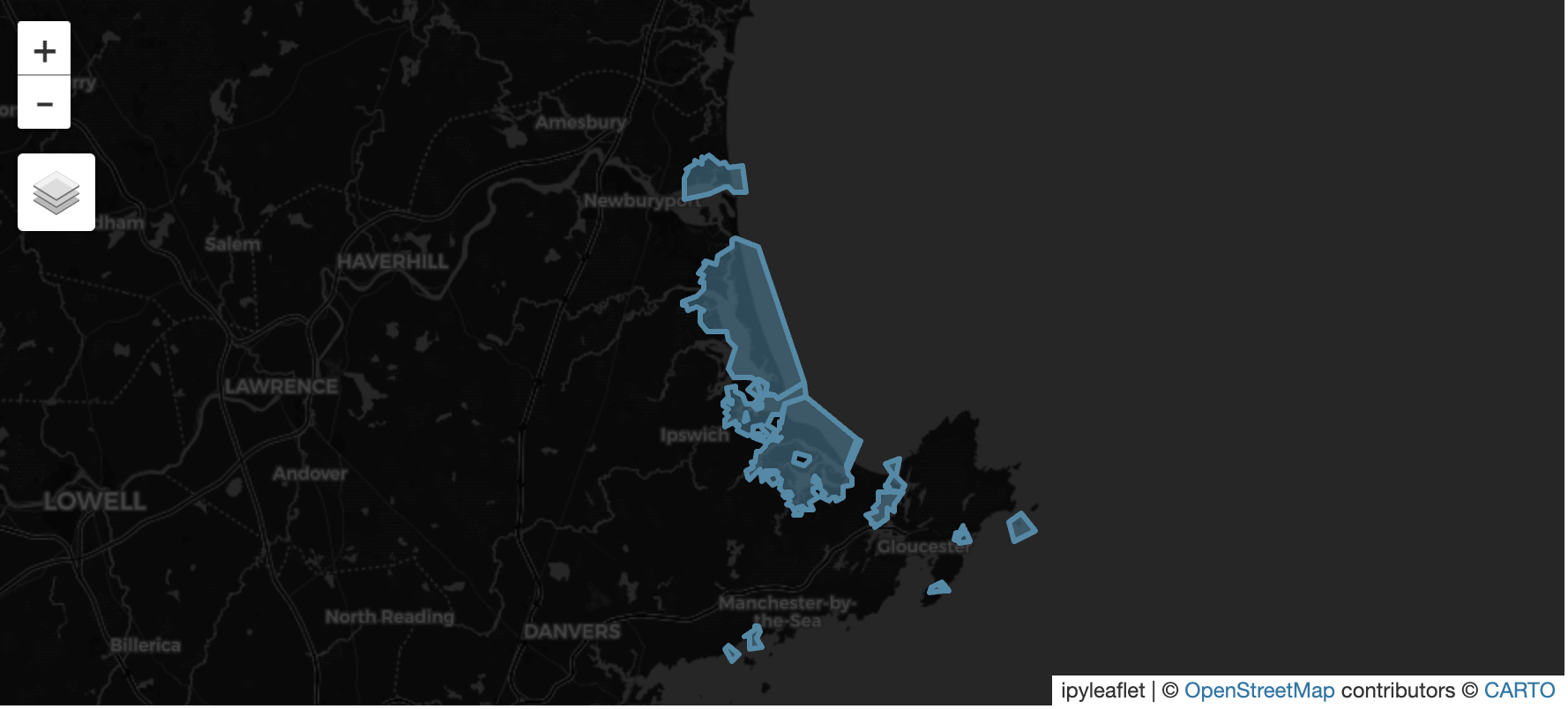